Why We Replaced Redux by React Query
In the journey of front-end development, choosing the right state management solution can significantly impact the efficiency and maintainability of your application. Two popular libraries, Redux and React Query, have emerged as key players in this domain. Redux has long been the default choice for managing application state in React applications, but React Query offers a fresh and potent alternative.
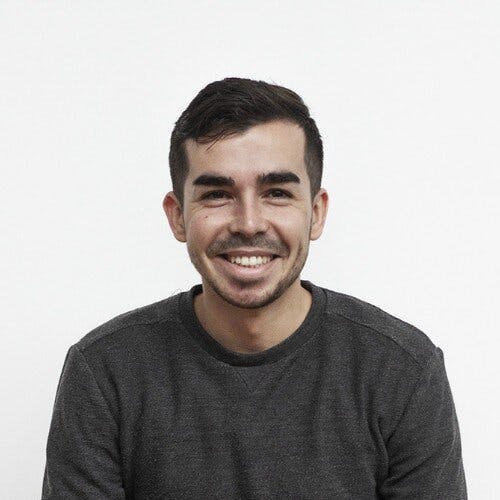
Rémi Bonnet
November 14, 2023 · 7 min read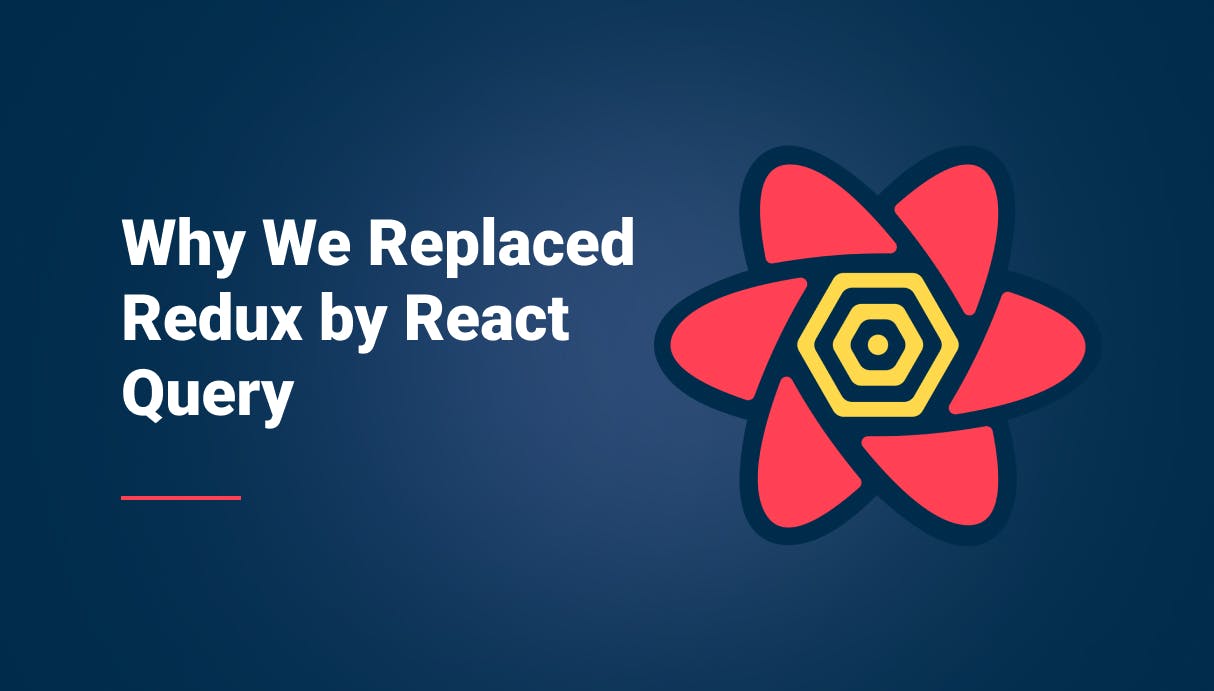
In this article, we embark on a journey to share our firsthand experience and insights. We'll illustrate the compelling reasons that drove us to transition from Redux to React Query, emphasizing the advantages it brings to the table. Through a practical example, we'll demonstrate how React Query simplifies and modernizes our state management approach.
Whether you're an experienced Redux user looking for a modern solution or new to state management choices, this article is a guide to help you make an informed decision to enhance your React application's state management.
TL;DR: here are our key benefits of React Query over Redux:
- Simplifies state management with automatic handling of error, loading, and fetching states.
- Reduces code complexity by eliminating the need for manual state management.
- Enhances maintainability and debugging with more readable and straightforward code.
- Avoids complex concepts like reducers, selectors, thunks, and dispatch, making it user-friendly and efficient.
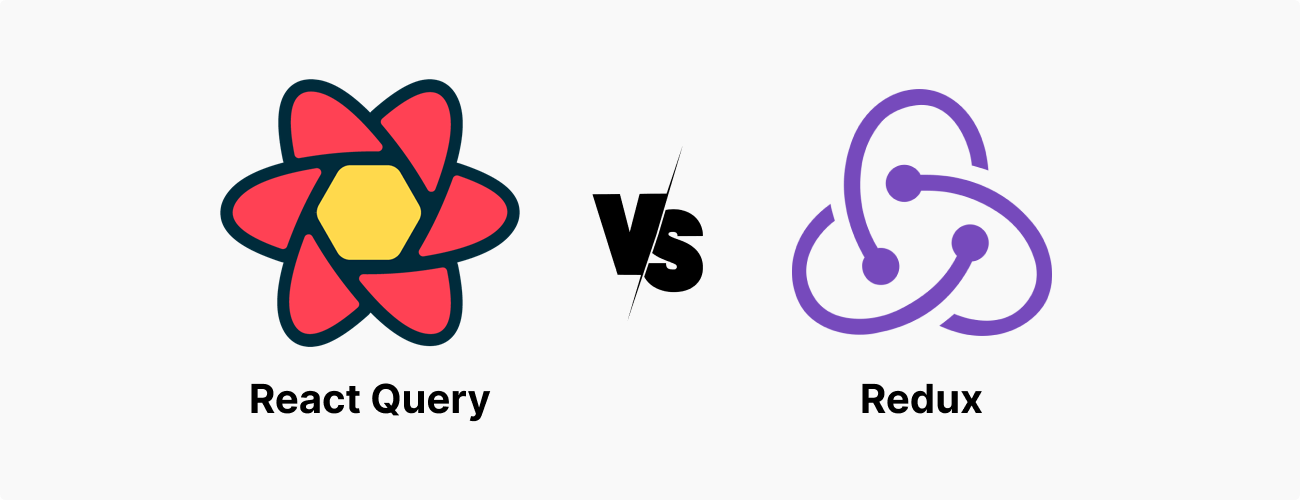
#Server State vs. Client State
The choice depends on your application’s requirements, with React Query excelling in server data scenarios, while Redux is robust for client-side state management. If you’re looking for additional resources on this topic, you can read Client vs. Server and replace client-side.
In contrast, Redux focuses on client-side state, centralizing application state management within the Client. Redux is suitable when you need precise control over the client-side state.
The choice depends on your application’s requirements, with React Query excelling in server data scenarios, while Redux is robust for client-side state management. If you’re looking for additional resources on this topic, you can read Client vs. Server and replace client-side.
#4 Compelling Advantages of React Query Over Redux
React Query offers several advantages, making it an attractive choice for modernizing our state management:
#Simplified Asynchronous Data Handling
Redux often requires writing verbose asynchronous action creators and middleware to manage data fetching and updating. React Query simplifies this process by providing a clean and straightforward way to handle asynchronous data fetching. With React Query, you can easily define queries and mutations, abstracting away much of the complexity that Redux typically involves.
#Efficient Data Caching
One of React Query's standout features is its built-in caching mechanism. It intelligently stores and updates data on the client side, reducing the need for redundant network requests. In contrast, Redux relies heavily on manual caching implementations, which can be error-prone and less efficient.
#Real-time Data Reactivity
While Redux primarily focuses on managing the application's global state, React Query extends its capabilities by offering real-time data reactivity out of the box. This means that when data changes on the server, React Query can automatically update your UI without the need for additional setup. Redux often requires the integration of libraries like Redux-Saga or Redux-Thunk to achieve similar functionality.
#Ecosystem and Community Support
React Query has gained significant momentum in the React community, and it continues to receive active development and support. As a result, you can benefit from an extensive ecosystem of plugins and community-contributed integrations. Redux, while still widely used, has seen a shift in popularity toward more simple and modern alternatives like React Query.
#Transitioning from Redux to React Query
We'll start by presenting a simplified Redux implementation, focusing on the key aspects rather than the full architectural details.
Firstly, let's take a look at the slice responsible for fetching User Account Information:
import { createSlice, createAsyncThunk } from '@reduxjs/toolkit'
import { type AccountInfo, AccountInfoApi } from 'qovery-typescript-axios' // Import your API or service for user data here
interface UserState {
data: AccountInfo | null
loading: boolean
error: string | null
}
const accountApi = new AccountInfoApi()
// Create an asynchronous action to perform the request
export const fetchAccountInformation = createAsyncThunk<AccountInfo>(
'user/fetchAccountInformation',
async () => {
const result = await accountApi.getAccountInformation()
return result
}
)
export const initialUserState: UserState = {
data: null, // Initialize user data to null
loading: false,
error: null,
}
// Create a slice to manage user state
const userSlice = createSlice({
name: 'user',
initialState: initialUserState,
reducers: {},
extraReducers: (builder) => {
builder
.addCase(fetchAccountInformation.pending, (state) => {
state.loading = true;
})
.addCase(fetchAccountInformation.fulfilled, (state, action) => {
state.loading = false;
state.data = action.payload;
})
.addCase(fetchAccountInformation.rejected, (state, action) => {
state.loading = false;
state.error = action.error.message;
});
},
})
export default userSlice.reducer
// Export the action to reuse it in your components
export { fetchAccountInformation }
Now, let's consider a basic usage example within a custom component called UserProfile:
import React, { useEffect } from 'react'
import { useDispatch, useSelector } from 'react-redux'
import { fetchAccountInformation } from './user-slice.ts' // Import the action
function UserProfile() {
const dispatch = useDispatch()
const { data, loading, error } = useSelector((state) => state.user)
useEffect(() => {
// Dispatch the fetchAccountInformation action when the component mounts
dispatch(fetchAccountInformation())
}, [dispatch])
if (loading) {
return <p>Loading user information...</p>;
}
if (error) {
return <p>Error: {error}</p>;
}
if (!data) {
return <p>No user data available.</p>;
}
return (
<div>
<h1>User Profile</h1>
<p>Name: {data.name}</p>
</div>
)
}
export default UserProfile
Indeed, the Redux Slice approach can become verbose, and while using useEffect in this context is necessary, it introduces some complexity. However, in React Query, we can achieve the same functionality with remarkable simplicity. Take a look at how the Redux Slice code can be entirely replaced by the following concise React Query example:
export function useUserAccount() {
return useQuery({
queryKey: ['userAccount'],
queryFn: async () => {
const result = await accountApi.getAccountInformation()
return result.data;
},
})
}
With React Query, we not only eliminate the need for Redux Slice, but we also gain automatic management of loading, error handling, and data retrieval — all in just a few lines of code. This simplicity and efficiency are some of the key advantages that make the transition from Redux to React Query a compelling choice for state management in React applications.
You can use it on our UserProfile removing useEffect and Redux dispatch, selector, by this:
const { data, isError, isLoading } = useUserAccount()
Now, let's explore how to simplify data access with React Query and Query Key Factory while enhancing type safety. Let's get started!
#Improving type safety with React Query and Query Key Factory
To enhance development and ensure type safety, we'll utilize @lukemorales/query-key-factory. This library provides type-safe query key management for React Query, complete with auto-completion features, making our transition even more efficient and reliable (for further details, you can refer to the React Query documentation).
In this code, we’ll make the data access for retrieving user account information. While we won't cover all implementation details, this example demonstrates the creation of query keys and an asynchronous query function.
import { createQueryKeys } from '@lukemorales/query-key-factory'
import { AccountInfoApi } from 'qovery-typescript-axios'
const accountApi = new AccountInfoApi()
export const user = createQueryKeys('user', {
account: {
queryKey: null,
async queryFn() {
const result = await accountApi.getAccountInformation()
return result.data
},
},
})
To further improve our workflow, we’ll create a global component that merges all query keys for reuse with our hook:
import { useQuery } from '@tanstack/react-query'
import { queries } from './state-queries.ts'
export function useUserAccount() {
return useQuery({
...queries.user.account,
})
}
export default useUserAccount
With these improvements made using React Query, we can revisit our UserProfile example and simplify it:
import React, { useEffect } from 'react'
import { useUserAccount } from './use-user-account.ts'
function UserProfile() {
const { data, isError, isLoading } = useUserAccount()
if (isLoading) {
return <p>Loading user information...</p>;
}
if (isError) {
return <p>User error</p>;
}
if (!data) {
return <p>No user data available.</p>;
}
return (
<div>
<h1>User Profile</h1>
<p>Name: {data.name}</p>
</div>
);
}
export default UserProfile
With these enhancements through React Query and the Query Key Factory library, we've achieved the following benefits:
- Simplicity: Our code has become simpler and easier to read with React Query, as it automatically manages error, loading, and fetching states. This eliminates the need for manual state management, reducing code complexity and making maintenance and debugging simpler. React Query is user-friendly and avoids specific concepts like reducer, extraReducer, selector, thunk, and dispatch, making it straightforward to use.
- Efficiency: State management is smoother, allowing for more efficient resource utilization.
- Type Safety: By using TypeScript and query key management, we've reinforced type safety, reducing potential errors.
- Reusability: Query keys and custom hooks are easily reusable throughout the application, enhancing development productivity.
- Modernization: This transition represents a modern and streamlined approach to state management in React applications, keeping your codebase up to date with the latest practices and libraries.
#Conclusion
In conclusion, our transition from Redux to React Query, coupled with the Query Key Factory library, has been enlightening and rewarding. We've seen significant benefits in adopting a more modern approach to state management. While Redux has introduced an equivalent solution with RTK Query, addressing similar challenges, React Query remains the preferred, more simple and mature choice in the current landscape. It's for these reasons that we've chosen React Query for our state management needs.
Thank you for reading!
Your Favorite Internal Developer Platform
Qovery is an Internal Developer Platform Helping 50.000+ Developers and Platform Engineers To Ship Faster.
Try it out now!Your Favorite Internal Developer Platform
Qovery is an Internal Developer Platform Helping 50.000+ Developers and Platform Engineers To Ship Faster.
Try it out now!