Adding Elegant Shadows with React to Invite Users to Scroll
By keeping your content in a box with a reasonably small height, you risk keeping hidden a part of your content. Users may not know that they can scroll down. How do you make sure they don't miss anything? At Qovery, we love to find elegant and subtle designs that encourage users to better use our product. In this article, I will explain how I added a Shadow effect to invite our users to scroll down content. Let's go!
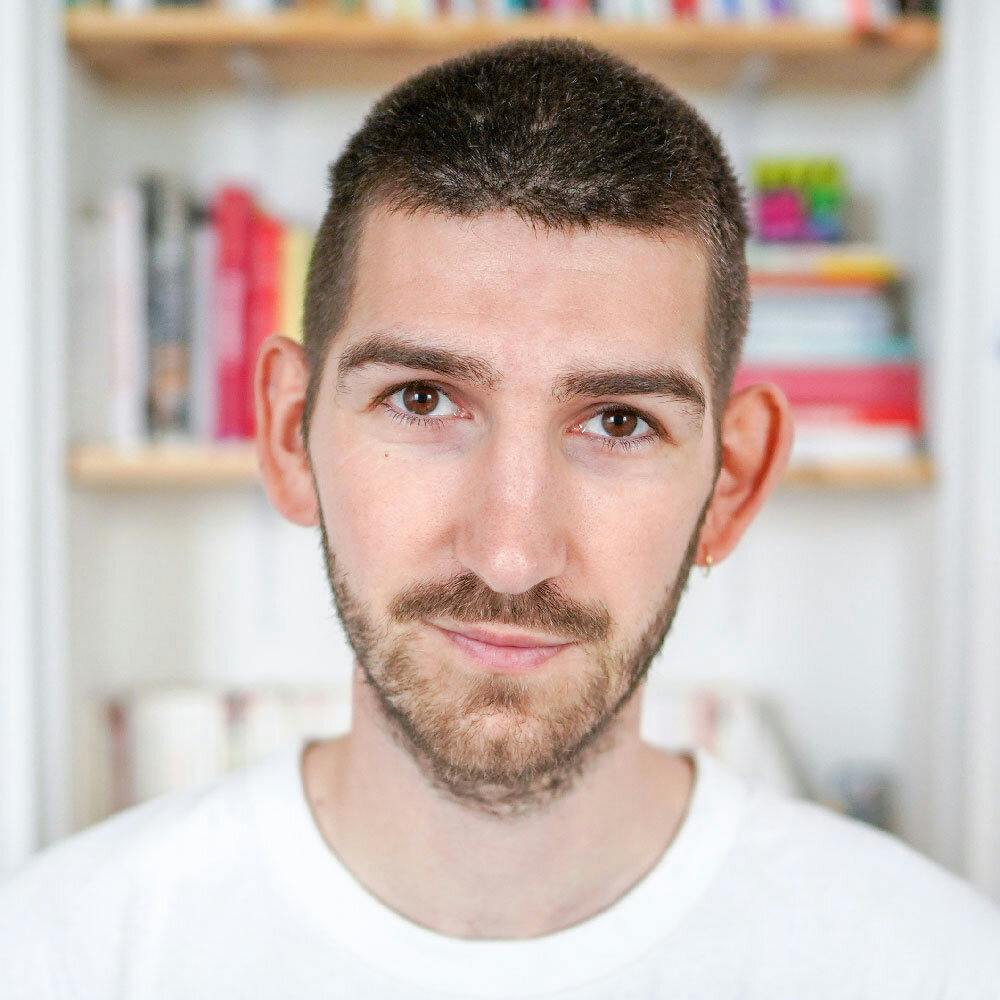
Benjamin Debon
December 26, 2022 · 5 min read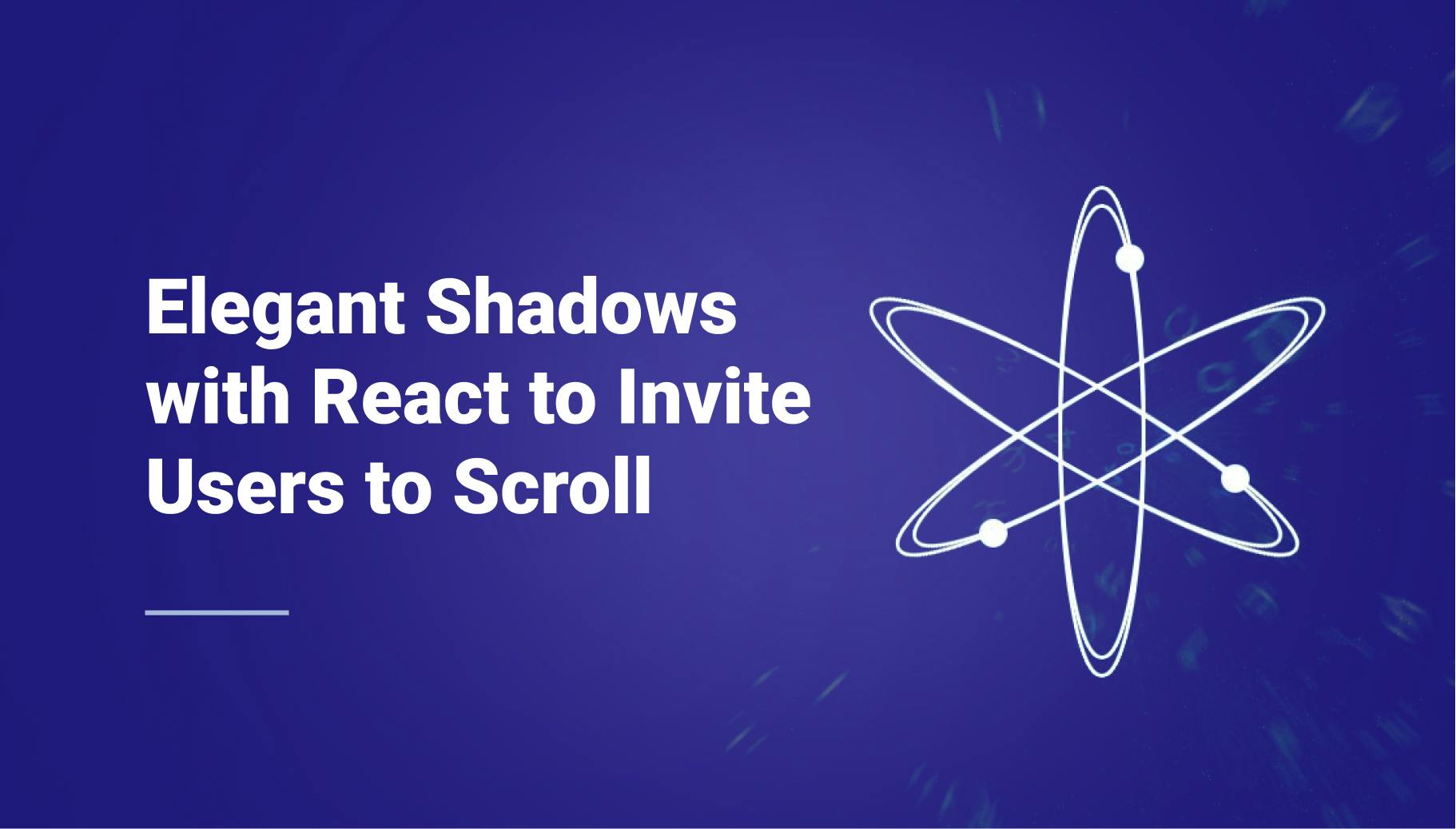
Before we start, let's quickly illustrate what we are talking about. Yes, this is what you see below. A simple shadow effect 🙂. (It's more complex than what it looks like - keep reading).
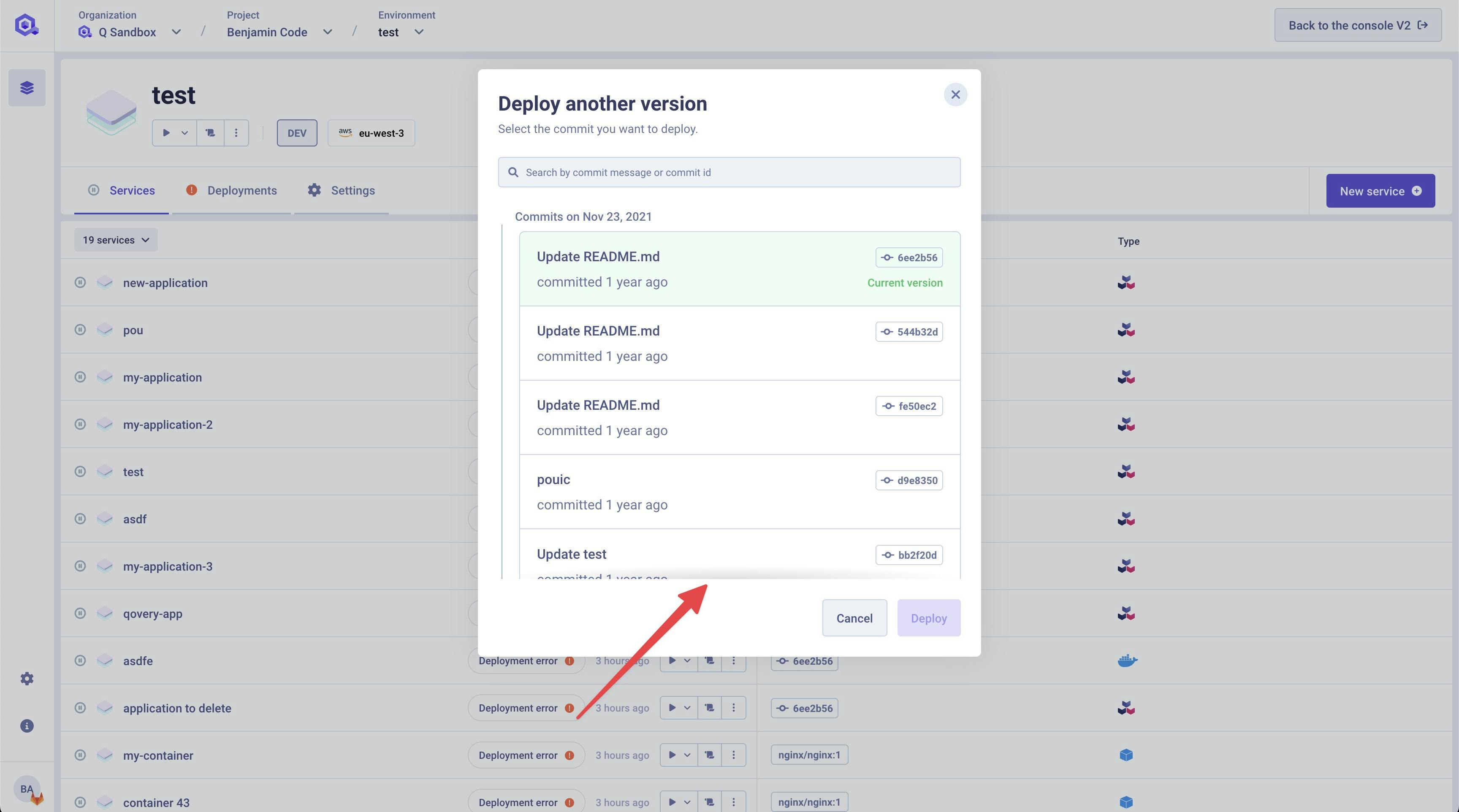
The thing is that adding this shadow can be a little bit tricky because you want it to be here at the beginning. Then if you scroll, you want to have it on top and bottom. And if you reach the bottom, you want to hide the bottom shadow.
After implementing it the first time with a bad developer experience on our v2, we have decided to redo it from scratch on our React v3.
In this article, you will learn how to do it and what we added to the common implementation you usually find everywhere on google.
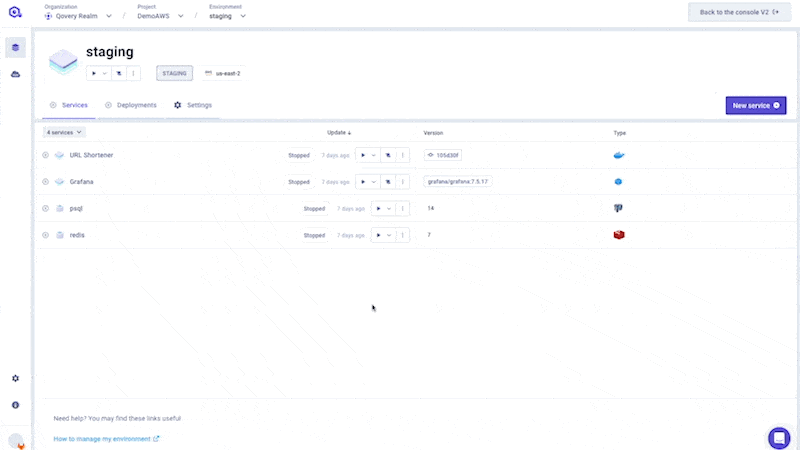
#A First Glimpse Into the Developer Experience
At the end of this article, you should have been able to use this component as quickly as this:
<ScrollShadowWrapper className="max-h-[440px]">
<ul>
<li>Your</li>
<li>Very</li>
<li>long</li>
<li>long</li>
<li>long</li>
<li>long</li>
<li>long</li>
<li>long</li>
<li>long</li>
<li>long</li>
<li>content</li>
</ul>
</ScrollShadowWrapper>
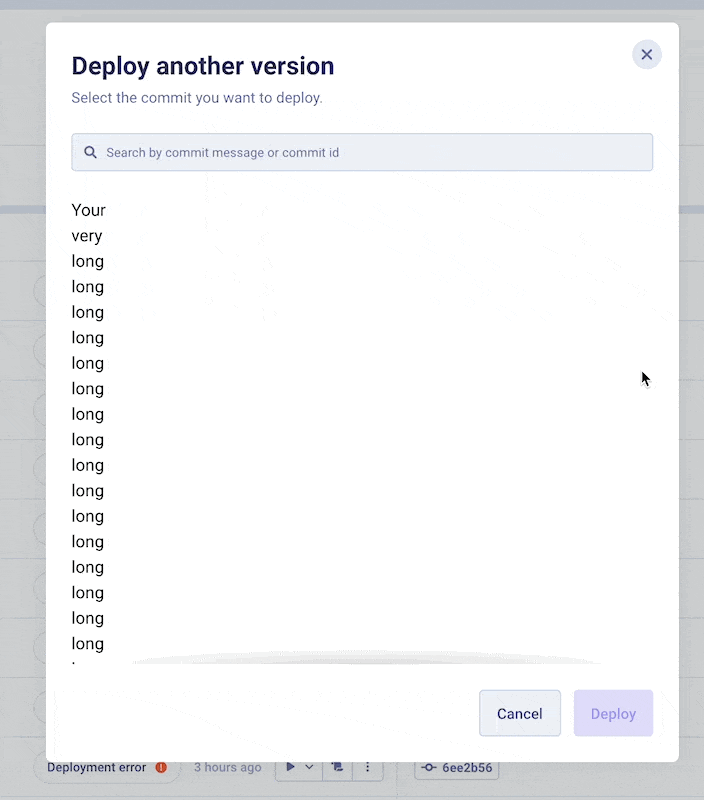
You have to wrap your content with the "<ScrollShadowWrapper>", giving it a max height; that’s it!
#What our Implementation Improves Compared to the One we Found Anywhere Else
Shout out to Marius Ibsen, that created a pretty elegant way to detect when we need the top and the bottom shadow. He documented that in his medium article.
The problem with his implementation is not in the detection but in the shadow itself. His shadows are done with "box-shadow inset", and the problem with the box shadows is that they are pretty ugly, and you don’t have any control over them.
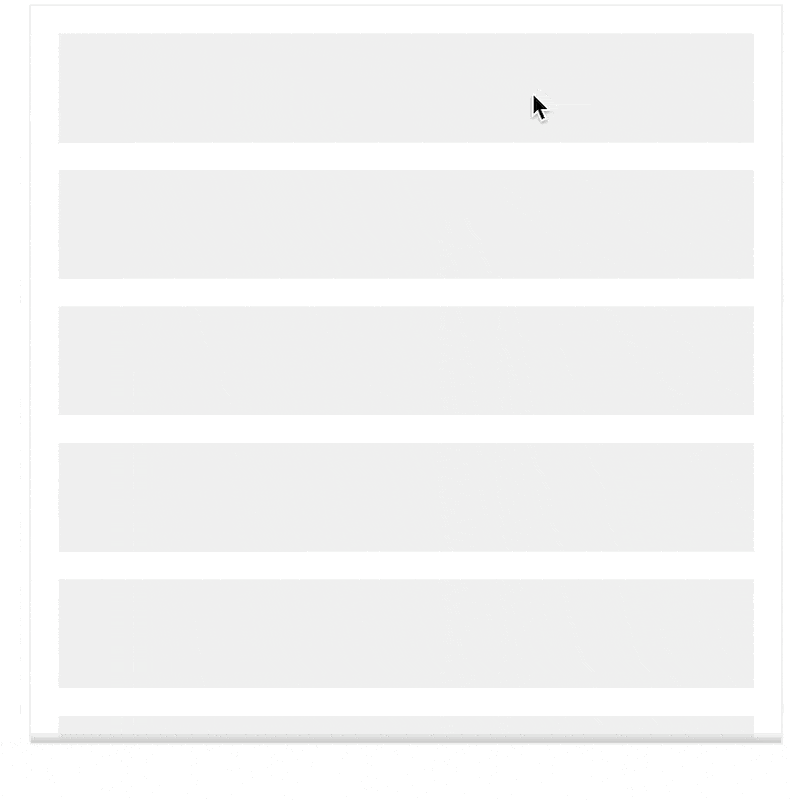
So instead of using a hook as he does, we use a WrapperComponent to embed all the improvements we want. And our improvements are on the count of 2.
One is about the shadows, but another, which we will cover a little bit later, provides an auto re-computation of the shadow presence when the content evolves. If the content gets bigger for any reason (async loading, filtering, …), the shadow presence must be re-asserted.
Our Own Beautiful Shadows
To implement the shadows, we used two div that stick to the top and the bottom of the wrapper with the "sticky" CSS property.
<div
ref={wrapperRef}
style={style}
className={`relative overflow-auto ${className}`}
onScroll={onScrollHandler}
>
{/* SHADOW TOP */}
<div
className={`sticky top-0 bg-scroll-shadow-up w-full h-4 -mb-4 transition-opacity duration-300 ${
getVisibleSides().top ? 'opacity-100' : 'opacity-0'
}`}
/>
{children}
{/* SHADOW BOTTOM */}
<div
className={`sticky bottom-0 bg-scroll-shadow-bottom w-full h-4 -mt-4 rotate-180 transition-opacity duration-300 ${
getVisibleSides().bottom ? 'opacity-100' : 'opacity-0'
}`}
/>
</div>
We use Tailwind in our example, but you can use whatever you want for your own implementation. As long as you set your blocks as "sticky" and, respectively "top-0" and "bottom-0"
#Another trick to keep the shadows on top of the content
By default, sticky keeps your block in the flow, so if your shadow is 16px tall, it will add 16px on top of your content, and you won’t have the desired effect; your shadow will be floating in the middle of nowhere instead of being on top of the content.
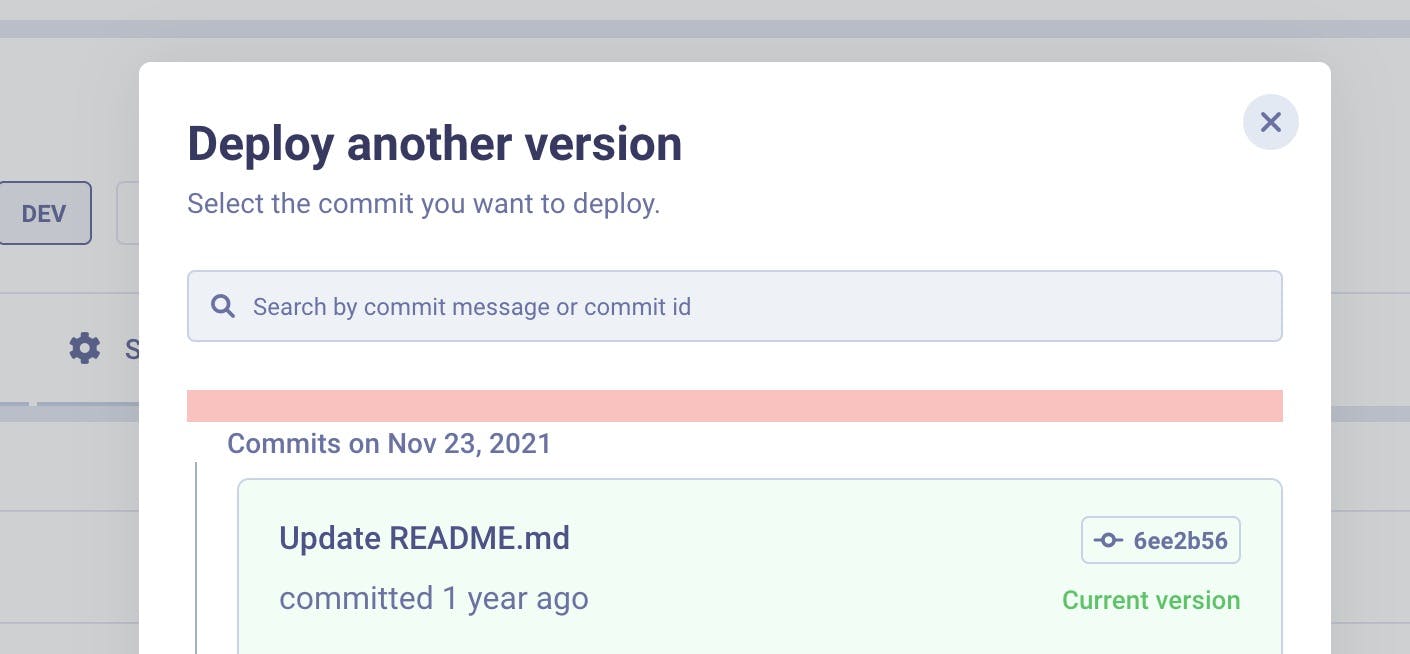
What you want to do, is to remove the size of the shadow from the flow, you want the shadow, to be on top of your content
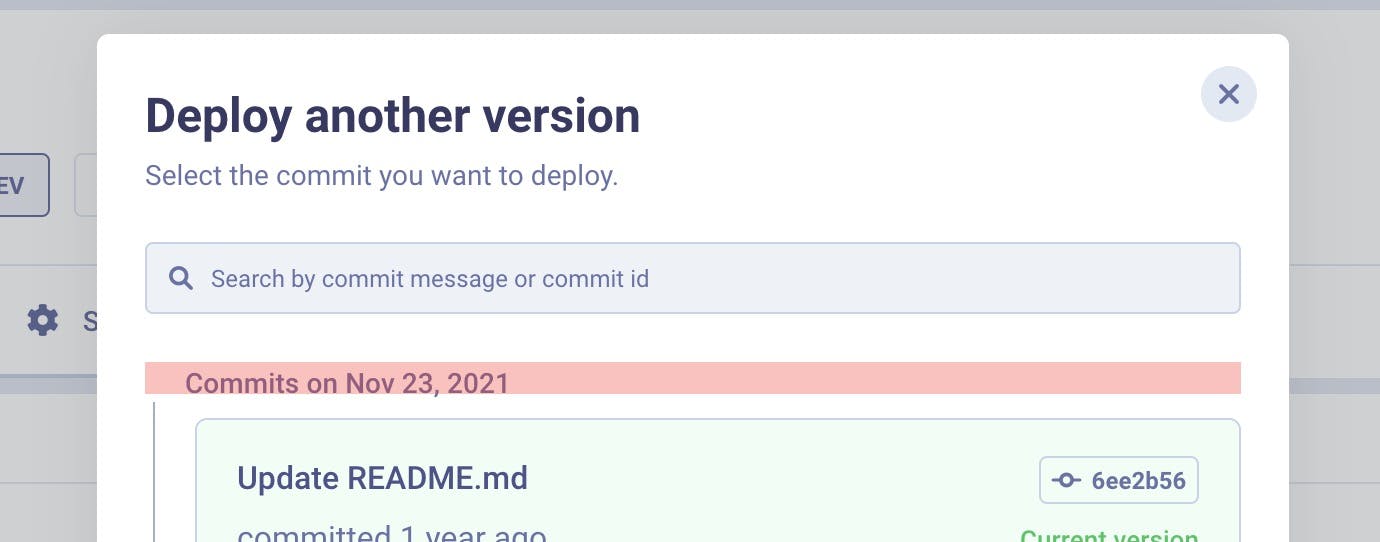
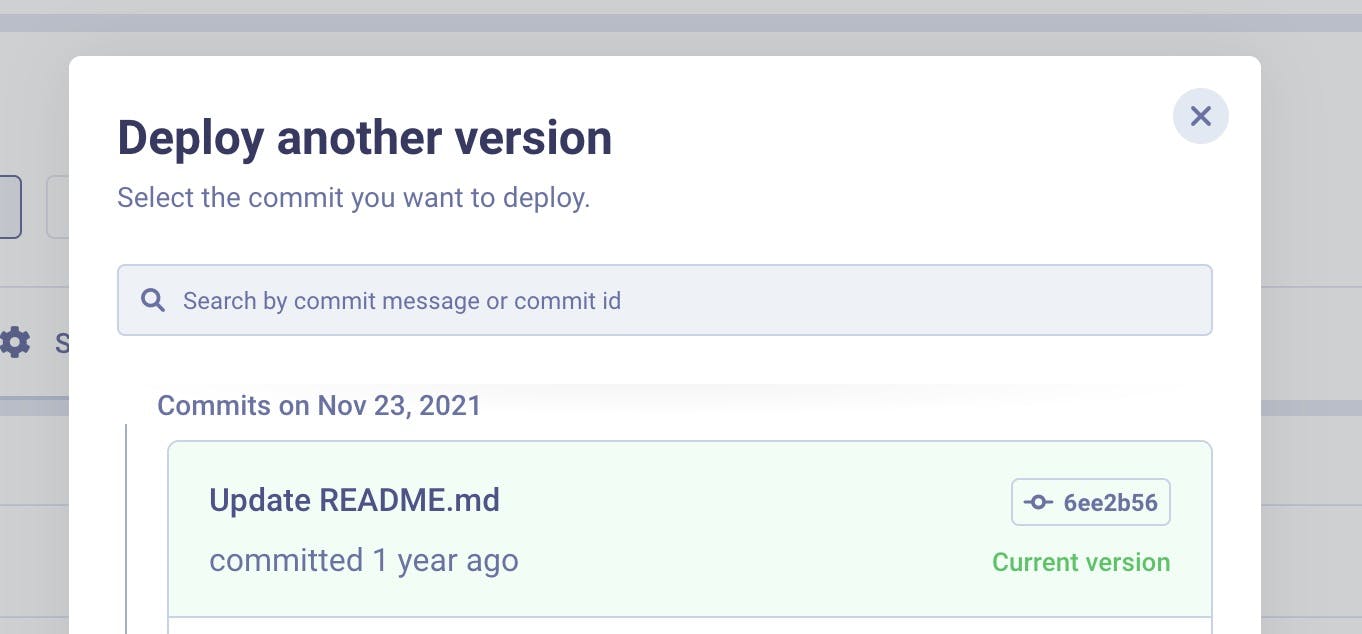
For this trick, you need to add a negative bottom margin to the shadow of the top and a negative top margin to the shadow of the bottom.

With that, you should be able to place your shadows correctly and give them the design you want.
#Activating the Shadow
To compute if your component must show the top shadow, the bottom one, or both at the same time, we re-used the technic of Marius because it was working super well (kudos to you).
export function ScrollShadowWrapper(props: ScrollShadowWrapperProps) {
const { children, className = '', style = {} } = props
const [scrollTop, setScrollTop] = useState(0)
const [scrollHeight, setScrollHeight] = useState(0)
const [clientHeight, setClientHeight] = useState(0)
const onScrollHandler = (event: React.WheelEvent<HTMLDivElement>) => {
setScrollTop(event.currentTarget.scrollTop)
setScrollHeight(event.currentTarget.scrollHeight)
setClientHeight(event.currentTarget.clientHeight)
}
const wrapperRef = useRef<HTMLDivElement>(null)
const getVisibleSides = (): { top: boolean; bottom: boolean } => {
const isBottom = clientHeight === scrollHeight - scrollTop
const isTop = scrollTop === 0
const isBetween = scrollTop > 0 && clientHeight < scrollHeight - scrollTop
return {
top: (isBottom || isBetween) && !(isTop && isBottom),
bottom: (isTop || isBetween) && !(isTop && isBottom),
}
}
return (
<div
ref={wrapperRef}
style={style}
className={`relative overflow-auto ${className}`}
onScroll={onScrollHandler}
>
{/*SHADOW TOP*/}
<div
className={`sticky top-0 h-4 -mb-4 bg-scroll-shadow-up w-full transition-opacity duration-300 ${
getVisibleSides().top || true ? 'opacity-100' : 'opacity-0'
}`}
/>
{children}
<div
className={`sticky bottom-0 bg-scroll-shadow-bottom w-full h-4 -mt-4 rotate-180 transition-opacity duration-300 ${
getVisibleSides().bottom || true ? 'opacity-100' : 'opacity-0'
}`}
/>
</div>
)
}
export default ScrollShadowWrapper
You listen to the scroll of your component and handle bit by the "onScrollHandler" function that saves the last scroll position and information about the scrollbar.
As it sets values via "useState", the component is being rendered, so the "getVisibleSides()" function is triggered again and says if yes or not, you have to show the top and bottom shadow.
That’s pretty much it.
But as I told you earlier, this won’t update the shadow visibility if your content height updates.
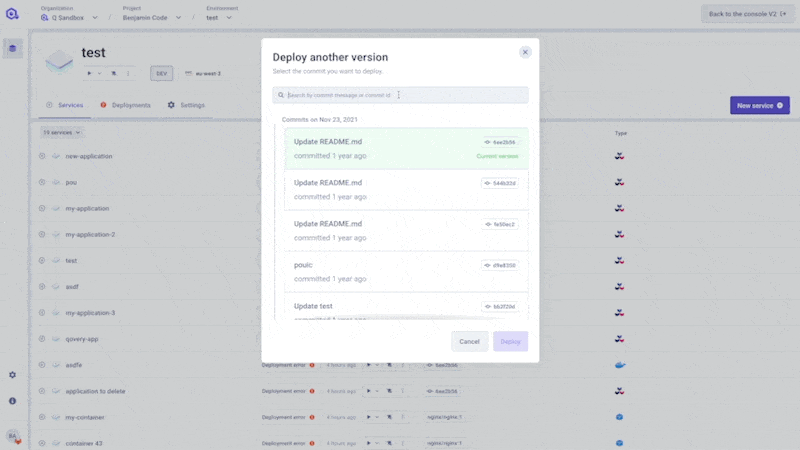
Fixing the Shadow if Content Evolves
The logic here is simple when the content height evolved, the height of our wrapper evolved. Still, no scroll events were triggered, so we are not recomputing anything, and the shadow stays in the state it was, even though we potentially don’t need it anymore.
We can easily fix that by resetting the values to the current one every time its height evolves.
This time, we can’t get the component through "event.currentTarget" because there is no event. This is why we have to use a ref.
const wrapperRef = useRef<HTMLDivElement>(null)
useEffect(() => {
const resetRefSizes = (ref: RefObject<HTMLDivElement>) => {
if (!ref.current) return
setScrollTop(ref.current.scrollTop)
setScrollHeight(ref.current.scrollHeight)
setClientHeight(ref.current.clientHeight)
}
resetRefSizes(wrapperRef)
}, [wrapperRef?.current?.clientHeight])
With that done, you are ready to go!
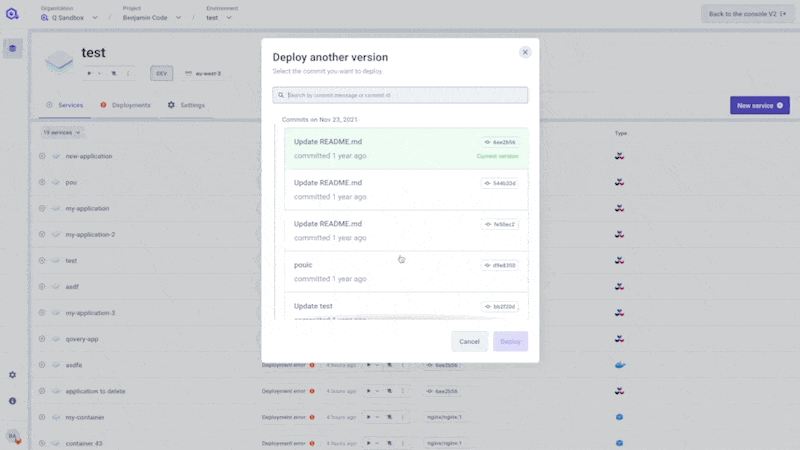
#Conclusion and Source Code
At Qovery, we love building our new interface in public. Our repository is open-source. You may then access our implementation and see the full source code here. You can also see how we implemented the unit tests for this not-quite-conventional thing to test.
We hope it will help you to reproduce this kind of neat effect on your product, and if many of you request it in the issues of our repository, we will maybe make an npm package for this component so that all of you can enjoy it even more efficiently.
Your Favorite DevOps Automation Platform
Qovery is a DevOps Automation Platform Helping 200+ Organizations To Ship Faster and Eliminate DevOps Hiring Needs
Try it out now!Your Favorite DevOps Automation Platform
Qovery is a DevOps Automation Platform Helping 200+ Organizations To Ship Faster and Eliminate DevOps Hiring Needs
Try it out now!